There can be some scenarios where you will want to convert ByteArray to a File like bytearray to bitmap or audio files or any other type of file in your Android App. Simply speaking a byte array is an array of bytes, a byte consists of 8 bit. Byte array can be use to store the collection of binary data like the contents of a file.
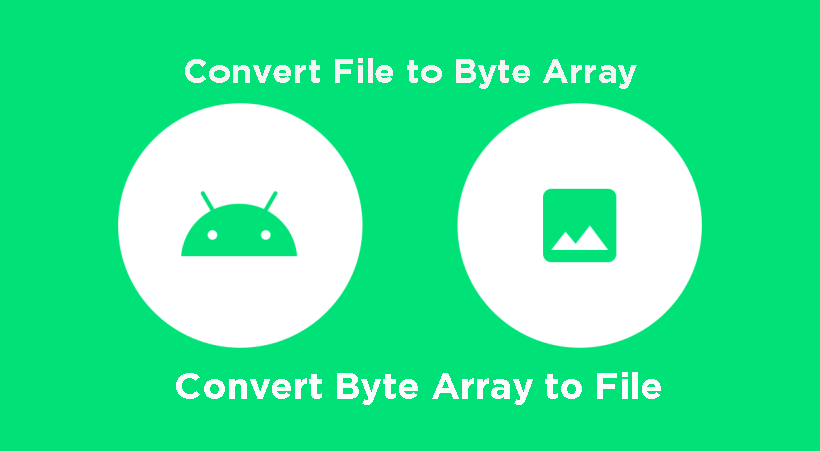
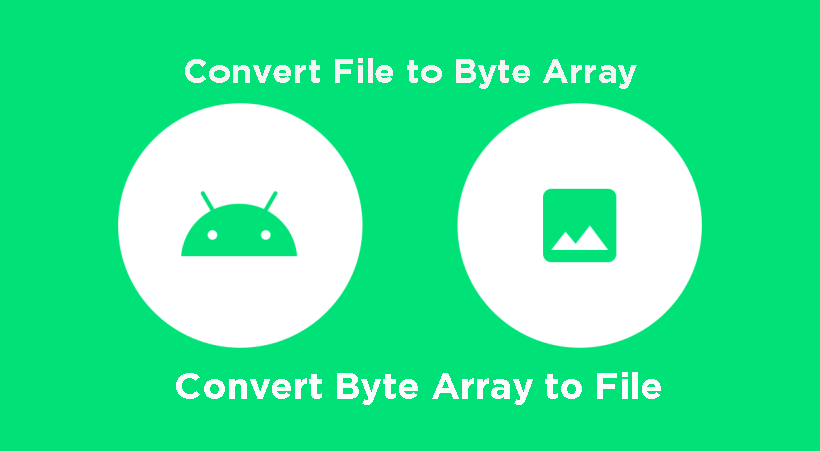
The following code shows how you can convert byte array to a file and a file to byte array in Android.
Converting File to Byte Array in Android
You can do this using input streams in Java like this:
public static byte[] getByteArrayFromAudio(String filePath) {
FileInputStream fis = null;
try {
fis = new FileInputStream(filePath);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
byte[] b = new byte[1024];
for (int readNum; (readNum = fis.read(b)) != -1; ) {
bos.write(b, 0, readNum);
}
return bos.toByteArray();
} catch (Exception e) {
Log.d("mylog", e.toString());
}
return null;
}
Converting Byte Array to File in Android
You can do this using buffered output streams in Java as shown below:
public static byte[] getByteArrayFromAudio(String filePath) {
FileInputStream fis = null;
try {
fis = new FileInputStream(filePath);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
byte[] b = new byte[1024];
for (int readNum; (readNum = fis.read(b)) != -1; ) {
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream(yourFile));
bos.write(fileBytes);
bos.flush();
bos.close();
For example, the code below shows how you can convert a bitmap to a byte array and vice-versa:
Bitmap to Byte Array:
public static byte[] getByteArray(Bitmap bitmap) {
ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();
bitmap.compress(Bitmap.CompressFormat.PNG, 0, byteArrayOutputStream);
return byteArrayOutputStream.toByteArray();
}
Byte Array to Bitmap
Bitmap bitmap = BitmapFactory.decodeByteArray(bitmapdata, 0, bitmapdata.length);
So this is how you can convert bitmap array to file or file array to bitmap in Android using Java, if you have any issues feel free to let me know in the comment section below.