Sometimes we need to load image from Android Studio’s assets folder, in this tutorial we’ll see how we can exactly load images from Assets folder in Android Studio, and set it to an ImageView.
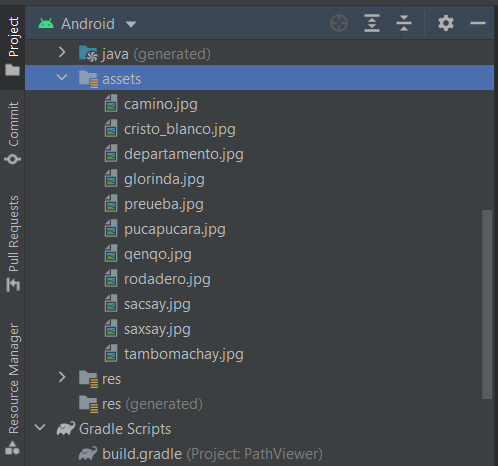
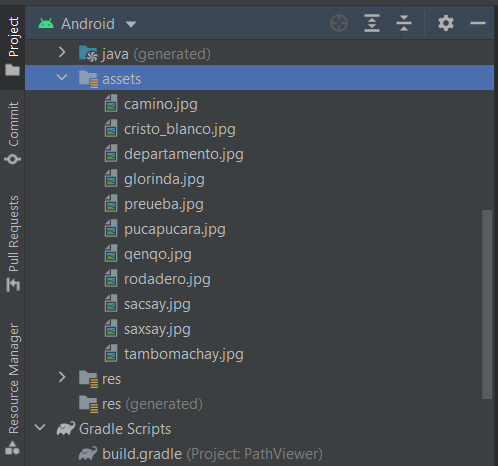
There are more than one ways to load image from the assets folder, but I’ll show you the most simplest one. Unlike the drawable folder, we cannot load image from assets by doing something like this: R.drawable.imagename, we need to read the image from a stream and create a drawable from the stream. After converting the stream to a drawable, we can then set this drawable to any imageview as we like.
Check the code below showing how you can load image from the assets folder:
Reading from Assets
try {
// get input stream
InputStream ims = context.getAssets().open(fileName);
// load image as Drawable
Drawable d = Drawable.createFromStream(ims, null);
imageView.setImageDrawable(d);
} catch (Exception ex) {
Log.d("mylog", "Error: " + ex.toString());
}
So we can simply load images using the code shown above, to improve the code and implement the DRY principle, you can put this code in a function and call this function wherever you want to load image from assets folder as shown below:
public Drawable getDrawableFromAssets(String fileName) throws IOException {
try {
// get input stream
InputStream ims = context.getAssets().open(fileName);
// load image as Drawable
Drawable d = Drawable.createFromStream(ims, null);
return d;
} catch (Exception ex) {
Log.d("mylog", "Error: " + ex.toString());
return null;
}
}
Now you can use this function wherever you’d like to load the image and still keep your code easy to read.
Setting Asset Drawable to ImageView
if (pathName.contains("camino")) {
holder.ivPath.setImageDrawable(getBitmapFromAssets("camino.jpg"));
} else if (pathName.contains("departamento")) {
holder.ivPath.setImageDrawable(getBitmapFromAssets("departamento.jpg"));
So you can easily load images from the assets folder in Android studio as shown above, if you run into any issue, feel free to let me know in the comments section below, and I’ll be happy to help you out! You can check more Android and Android Studio tutorials on our site!