I tutorial on how to create a PDF document from a layout or any XML view in Android Studio, I will walk you through the process of using the PdfDocument class to convert your XML views into PDF files. With this powerful functionality, you can generate professional-looking documents within your Android applications. Let’s dive in and explore the steps required to accomplish this. Before we get started, make sure you have the following pre-requisites:
- Android Studio installed on your system
- Basic knowledge of Android development and XML layout design
If you are a visual learner, you can find the full video tutorial here:
Create the XML Layout to convert to PDF
Begin by creating a new Android project in Android Studio. Choose an appropriate project name and configure the necessary settings for your application. Design your XML layout file with the desired elements, such as TextViews, ImageViews, and other components. Customize the layout according to your requirements. For the sake of this tutorial, we have use the following XML (main_activity.xml):
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:orientation="vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="16dp"
android:text="Learn to Create PDF"
android:textSize="24sp"
android:textStyle="bold" />
<Button
android:id="@+id/btnCreatePdf"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Create PDF" />
<Button
android:id="@+id/btnXMLToPDF"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Convert XML View to PDF" />
</LinearLayout>
This is what our layout looks like. Later on we will be converting this layout itself to PDF:
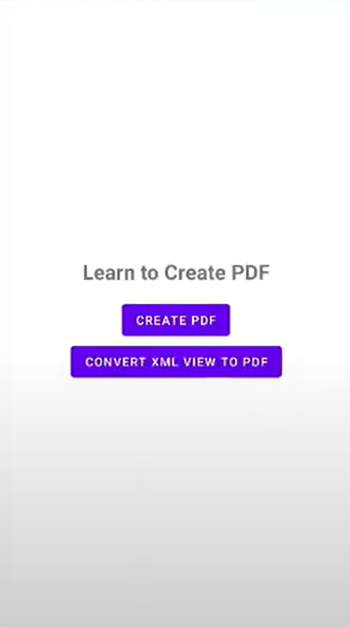
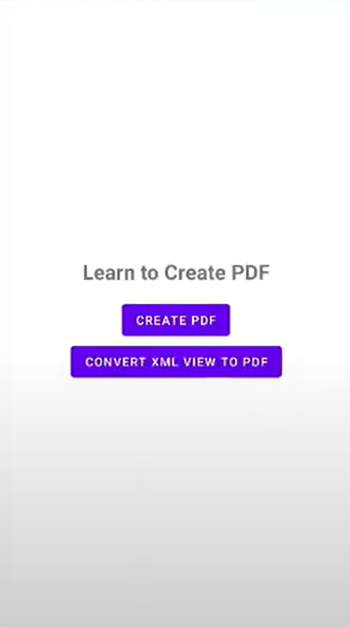
Creating the Main Activity to Generate PDF
Create an activity, we have called it main activity, this activity have the XML layout we created above as it’s content view. And we will create two functions.
- createPDF(): This function will be used to create PDF file and write text to it. We will use canvas and the function provided by canvas called: canvas.drawText() to write text to the PDF file
- convertXMLtoPDF(): This function will be used to convert the xml layout we created above(‘main_activity.xml’) to PDF and save it to local storage.
So once you’ve set the ContentView and added references to the views in the XML above, this is what the activity looks like:
public class MainActivity extends AppCompatActivity {
Button btnCreatePDF;
Button btnXMLtoPDF;
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main_activity);
askPermissions();
btnCreatePDF = findViewById(R.id.btnCreatePdf);
btnCreatePDF.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
createPDF();
}
});
btnXMLtoPDF = findViewById(R.id.btnXMLToPDF);
btnXMLtoPDF.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
convertXmlToPdf();
}
});
}
}
Note that we have not created the two functions convertXmlToPdf and createPDF as of now. We will implement those methods in the next steps.
You may also like: Download Any PDF from URL
Asking Write Permission to Store PDF Locally
In order to save the save the PDF to local storage of android device, we need to ask for runtime permission starting android version 6 and highter. So let’s go ahead and create a function that asks for permission, and add the function in onCreate of our main activity:
private void askPermissions() { ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE}, REQUEST_CODE); }
Also note the variable REQUEST_CODE. In the beginning of MainActivity, above onCreate. Add the following line:
final static int REQUEST_CODE = 1232;
Generating PDF using PDFDocument
Now let’s get to the good stuff, this is the part where we will implement two functions that will generate PDF. One will write Hello World to the PDF, and another function will convert XML Layout to PDF. Let’s jump into it:
Creating PDF and Writing to it
Let’s first look at the whole thing and then we will break it down so that you can understand it better. Here’s what the whole function looks like:
private void createPDF() {
PdfDocument document = new PdfDocument();
PdfDocument.PageInfo pageInfo = new PdfDocument.PageInfo.Builder(1080, 1920, 1).create();
PdfDocument.Page page = document.startPage(pageInfo);
Canvas canvas = page.getCanvas();
Paint paint = new Paint();
paint.setColor(Color.RED);
paint.setTextSize(42);
String text = "Hello, World";
float x = 500;
float y = 900;
canvas.drawText(text, x, y, paint);
document.finishPage(page);
File downloadsDir = Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_DOWNLOADS);
String fileName = "example.pdf";
File file = new File(downloadsDir, fileName);
try {
FileOutputStream fos = new FileOutputStream(file);
document.writeTo(fos);
document.close();
fos.close();
Toast.makeText(this, "Written Successfully!!!", Toast.LENGTH_SHORT).show();
} catch (FileNotFoundException e) {
Log.d("mylog", "Error while writing " + e.toString());
throw new RuntimeException(e);
} catch (IOException e) {
throw new RuntimeException(e);
}
}
PdfDocument document = new PdfDocument();
This line creates an instance of the PdfDocument class, which represents the PDF document we want to generate.PdfDocument.PageInfo pageInfo = new PdfDocument.PageInfo.Builder(1080, 1920, 1).create();
Here, we create a PageInfo object using the Builder pattern. We specify the page dimensions (width: 1080, height: 1920) and the page number (1) for the PDF document.PdfDocument.Page page = document.startPage(pageInfo);
This line starts a new page in the PdfDocument using the PageInfo object we created. The returned Page object represents the current page in the document.Canvas canvas = page.getCanvas();
We obtain a Canvas object from the Page object. The Canvas is a 2D drawing surface where we can draw graphics and text.Paint paint = new Paint();
We create a Paint object that holds the style and color information for drawing.paint.setColor(Color.RED);
paint.setTextSize(42);
Here, we set the color of the paint to red, and set the text size of the paint to 42 pixels.String text = "Hello, World";
float x = 500;
float y = 900;
Here we define the text that we want to draw on the canvas. Then we set the x-coordinate of the starting point of the text on the canvas and we set the y-coordinate of the starting point of the text on the canvas.canvas.drawText(text, x, y, paint);
This line draws the specified text on the canvas at the given coordinates using the paint object.document.finishPage(page);
We finish the current page in the document, indicating that we have completed drawing on it.File downloadsDir = Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_DOWNLOADS);
We get the path to the Downloads directory on the external storage.String fileName = "example.pdf";
We specify the name of the PDF file.File file = new File(downloadsDir, fileName);
We create a File object representing the PDF file with the specified directory and filename.FileOutputStream fos = new FileOutputStream(file);
We create a FileOutputStream to write the PDF document to the file.document.writeTo(fos);
We write the contents of the PdfDocument to the FileOutputStream.document.close();
fos.close();
We close the PdfDocument, indicating that we have finished working with it and we close the FileOutputStream.Toast.makeText(this, "Written Successfully!!!", Toast.LENGTH_SHORT).show();
Finally, we display a toast message indicating that the PDF has been written successfully.
Congratulations! You’ve create your first PDF file from your android app! Now you can create PDF files, and write content to it using canvas. Now let’s see how you can convert an Android View or XML Layout to PDF.
Converting View or XML Layout to PDF
So now that we can draw text to a canvas, and download the PDF to a folder in local storage, let’s see how we can convert XML view or layout to PDF file. As earlier, let’s see the whole code for first:
public void convertXmlToPdf() {
// Inflate the XML layout file
View view = LayoutInflater.from(this).inflate(R.layout.main_activity, null);
DisplayMetrics displayMetrics = new DisplayMetrics();
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.R) {
this.getDisplay().getRealMetrics(displayMetrics);
} else
this.getWindowManager().getDefaultDisplay().getMetrics(displayMetrics);
view.measure(View.MeasureSpec.makeMeasureSpec(displayMetrics.widthPixels, View.MeasureSpec.EXACTLY),
View.MeasureSpec.makeMeasureSpec(displayMetrics.heightPixels, View.MeasureSpec.EXACTLY));
Log.d("mylog", "Width Now " + view.getMeasuredWidth());
view.layout(0, 0, displayMetrics.widthPixels, displayMetrics.heightPixels);
// Create a new PdfDocument instance
PdfDocument document = new PdfDocument();
// Obtain the width and height of the view
int viewWidth = view.getMeasuredWidth();
int viewHeight = view.getMeasuredHeight();
// Create a PageInfo object specifying the page attributes
PdfDocument.PageInfo pageInfo = new PdfDocument.PageInfo.Builder(viewWidth, viewHeight, 1).create();
// Start a new page
PdfDocument.Page page = document.startPage(pageInfo);
// Get the Canvas object to draw on the page
Canvas canvas = page.getCanvas();
// Create a Paint object for styling the view
Paint paint = new Paint();
paint.setColor(Color.WHITE);
// Draw the view on the canvas
view.draw(canvas);
// Finish the page
document.finishPage(page);
// Specify the path and filename of the output PDF file
File downloadsDir = Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_DOWNLOADS);
String fileName = "exampleXML.pdf";
File filePath = new File(downloadsDir, fileName);
try {
// Save the document to a file
FileOutputStream fos = new FileOutputStream(filePath);
document.writeTo(fos);
document.close();
fos.close();
// PDF conversion successful
Toast.makeText(this, "XML to PDF Conversion Successful", Toast.LENGTH_LONG).show();
} catch (IOException e) {
e.printStackTrace();
// Error occurred while converting to PDF
}
}
The code above demonstrates the process of converting an XML layout file to a PDF document in Android using the PdfDocument class. Now let’s try to understand the code line by line:
View view = LayoutInflater.from(this).inflate(R.layout.main_activity, null);
This line inflates the XML layout file (specified byR.layout.main_activity
) into a View object.DisplayMetrics displayMetrics = new DisplayMetrics();
Here, a DisplayMetrics object is created to hold the display metrics of the device.if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.R) {
this.getDisplay().getRealMetrics(displayMetrics);
} else
this.getWindowManager().getDefaultDisplay().getMetrics(displayMetrics);
This conditional statement checks the Android SDK version. If the SDK version is equal to or higher than Android R, it uses thegetDisplay().getRealMetrics(displayMetrics)
method to obtain the real metrics of the display. Otherwise, it usesgetWindowManager().getDefaultDisplay().getMetrics(displayMetrics)
to get the display metrics.view.measure(View.MeasureSpec.makeMeasureSpec(displayMetrics.widthPixels, View.MeasureSpec.EXACTLY), View.MeasureSpec.makeMeasureSpec(displayMetrics.heightPixels, View.MeasureSpec.EXACTLY))
;
This line measures the dimensions of the View by calling themeasure()
method. It usesView.MeasureSpec.makeMeasureSpec()
to create the measurement specifications for width and height, which are set to match the display metrics obtained earlier.view.layout(0, 0, displayMetrics.widthPixels, displayMetrics.heightPixels);
Thelayout()
method is called on the View to set its position and dimensions. Here, it sets the View’s position to (0, 0) and its dimensions to match the display metrics.PdfDocument document = new PdfDocument();
An instance of the PdfDocument class is created, representing the PDF document to be generated.int viewWidth = view.getMeasuredWidth();
int viewHeight = view.getMeasuredHeight();
The width and height of the measured View are obtained.PdfDocument.PageInfo pageInfo = new PdfDocument.PageInfo.Builder(viewWidth, viewHeight, 1).create();
A PageInfo object is created using the Builder pattern. It specifies the width, height, and page number (1) for the PDF document.PdfDocument.Page page = document.startPage(pageInfo);
This line starts a new page in the PdfDocument using the PageInfo object. The returned Page object represents the current page in the document.Canvas canvas = page.getCanvas();
A Canvas object is obtained from the Page object. The Canvas is a 2D drawing surface on which we can draw graphics and text.Paint paint = new Paint();
paint.setColor(Color.WHITE);
A Paint object is created to define the style and color for drawing. Here, the paint color is set to white.view.draw(canvas);
The View is drawn on the canvas using thedraw()
method, which renders the View’s visual representation onto the canvas.document.finishPage(page);
The current page in the document is finished, indicating that we have completed drawing on it.File downloadsDir = Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_DOWNLOADS);
String fileName = "exampleXML.pdf";
File filePath = new File(downloadsDir, fileName);
The path and filename for the output PDF file are specified. Here, it is set to the Downloads directory with the filename “exampleXML
Conclusion – Creating PDF from View in Android Studio
In conclusion, this tutorial has demonstrated how to convert an XML layout or any XML view into a PDF document in Android Studio. By utilizing the PdfDocument class and the Canvas API, we were able to create a PDF representation of the XML layout or view.
Remember to handle any necessary permissions and make sure to test your implementation on different devices and screen sizes to ensure compatibility and a smooth user experience.
Let me know in case you run into any issues, Cheers!